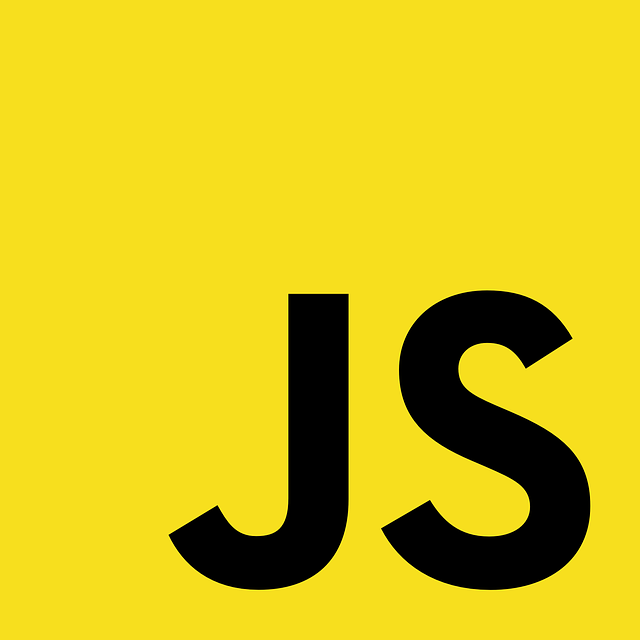
이벤트 버블링(Bubbling)
특정 영역에서 이벤트가 발생했을 때 이벤트가 해당 영역 상위로 전파되는 경우를 말한다.
1. html 구조 와 js에서 이벤트 만들기(버블링 예제)
<div id="first">
<h1>first</h1>
<div id="second">
<h1>second</h1>
<div id="third">
<h1>third</h1>
</div>
</div>
</div>
const first = document.querySelector('#first');
const second = document.querySelector('#second');
const third = document.querySelector('#third');
first.addEventListener('click', function(){
console.log('first');
})
second.addEventListener('click', function(){
console.log('second');
})
third.addEventListener('click', function(){
console.log('third');
})
html 구조는 first div > second div > third div 구조 다.(부모와 자식 구조로 생성)
first가 최상위 부모가 된다.
다음 js으로
first, second, third에 각각 click이벤트를 걸어주고 click 할 때 conosle을 출력하게 한다.
이후, third에 클릭을 한다.
2. third 클릭 했을 때(버블링)
third영역을 클릭 해서 console에 third만 출력될 것 같지만
console에 출력된 것을 보면 third → second → first 순서로 출력된다.
third의 click이벤트만 발생하는게 아니라 second, first 이벤트도 같이 발생된다.
3. second클릭했을 때(버블링)
second영역을 클릭하게 되면 second → first 순서로 출력된다.
클릭한 영역에 이벤트만 발생하는 게 아니라
이렇게 이벤트가 상위 요소로 전파되는 방식을 버블링이라고 한다.
이벤트 캡처(Capture)
이벤트 캡처는 이벤트 버블링과 반대 방향으로 진행되는 이벤트 전파 방식이다.
1. 캡쳐 이벤트 설정하기
const first = document.querySelector('#first');
const second = document.querySelector('#second');
const third = document.querySelector('#third');
first.addEventListener('click', function(){
console.log('first');
},true)
second.addEventListener('click', function(){
console.log('second');
},true)
third.addEventListener('click', function(){
console.log('third');
},true)
이벤트 캡처를 하기 위해서는 addEventListener() 안에 true를 설정해준다.
기본값이 false(버블링)이다.
2. third 클릭했을 때(캡처)
third를 클릭했을 때 first → second → third 순서로 출력된다.
버블링과 반대 방향으로 이벤트가 전파되고 실행된다.
3. second클릭했을 때(캡처)
second를 클릭했을 때 first → second 순서로 출력된다.
이렇게 캡처는 이벤트 전파가 상위에서 하위로 전파되고 실행된다.
이벤트 전파 막기
1. third에서 이벤트 막기
const first = document.querySelector('#first');
const second = document.querySelector('#second');
const third = document.querySelector('#third');
first.addEventListener('click', function(){
console.log('first');
})
second.addEventListener('click', function(){
console.log('second');
})
third.addEventListener('click', function(event){
event.stopPropagation();
console.log('third');
})
third.addEventListener('click', function(event){
event.stopPropagation();
console.log('third');
})
event.stopPropagation()를 사용하여 third에서 이벤트 전파를 막고 third에 클릭해본다.
위와 같이 콘솔에는 third만 출력된다.
third에서 second → first로 이벤트가 전파되지 않고 third에 이벤트만 실행되기 때문이다.
만약, 이상태에서 second를 클릭해보면 second → first 순서로 이벤트가 실행된다.
third에서만 이벤트를 막았을 뿐 second에서는 이벤트를 막지 않아 상위로 전파가 되기 때문이다.
이렇게 event.stopPropagation()를 사용하여 이벤트 전파를 제어 할 수 있다.
이벤트 위임
'개발노트 > Javascript' 카테고리의 다른 글
(JS) map() (2019/10/23)(보충필요!!) (0) | 2019.10.23 |
---|---|
(JS) Closure 클로저 (2019/10/21)(보충필요!) (0) | 2019.10.21 |
[JS] Boolean 데이터 타입 (0) | 2019.10.15 |
(JS) filter() (2019/10/14)(보충필요!) (0) | 2019.10.14 |
(JS) 객체(Object) (2019/10/10) (0) | 2019.10.10 |
주니어 개발자의 성장 기록지
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!